API调用(webhook/sdk)
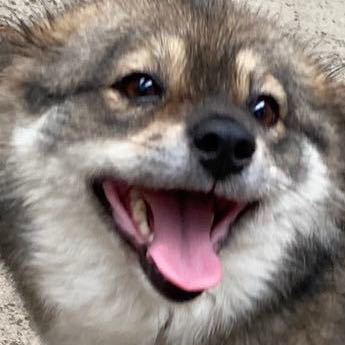
主要是如何去用webhook或者sdk去进行相应API的访问,包括了使用python、Java sdk以及Python、Java webhook进行相应的访问。
基本上所有的API调用都可以分为使用SDK和Url两种,这边以飞书开发作为案例。
飞书应用开发,个人版是无法使用的。公司开发相应的程序又需要权限,可以自己建立一个公司账户,虽然不经过认证,但也能使用相应的开发。
飞书应用主要有四种,网页版应用、小程序、机器人、工作台组件,还可以对一些开发的内部网站使用飞书进行登录。
具体开发的方式详见飞书开放平台开发文档,这里主要讲的是如何对webhook和飞书的sdk进行调用。
飞书API的调用
在调用相应的API前,要在开发者后台中创建企业自建应用,然后再开发文档的右上角有API调试台,进入后有相关的例子,以及参数的配置,只不过没有调用WebHook的例子,我这边也加上了相应的例子。
此机器人最终会发送消息,效果图如下:
一. Webhook
也就是使用url
发送数据包的方式去进行相应的访问,其中我们展示三种方式,分别是cmd中使用curl
,Python、Java制作数据包。
现在以飞书机器人发送消息API的调用作为示例进行演示,注意此样例需要获取相应的token和user_id,此样例中的两个参数是无法实际使用的。
下面是三种访问的代码:
Curl
此方法需要提前安装好
curl
命令。1
2
3
4
5
6
7
8curl -i -X POST 'https://open.feishu.cn/open-apis/im/v1/messages?receive_id_type=user_id' \
-H 'Authorization: Bearer t-g1042h8JMSNCGICKKETF' \
-H 'Content-Type: application/json' \
-d '{
"content": "{\"text\":\"2-17\"}",
"msg_type": "text",
"receive_id": "abcd1234"
}'Python Web
此方法需要先安装requests包,这个包是可以发送Http请求的。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25import requests
# 请求的URL
url = 'https://open.feishu.cn/open-apis/im/v1/messages?receive_id_type=user_id'
# 请求头
headers = {
'Authorization': 'Bearer t-g1042h8JMSNCGICKKETF',
'Content-Type': 'application/json'
}
# 请求数据
data = {
"content": "{\"text\":\"2-17\"}",
"msg_type": "text",
"receive_id": "abcd1234"
}
# 发送POST请求
response = requests.post(url, headers=headers, json=data)
# 打印响应状态码和内容
print("Status Code:", response.status_code)
print("Response Content:", response.text)Java Web
此方法使用的是
java.net.HttpURLConnection
数据包1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
public class FeishuPostExample {
private static final String URL = "https://open.feishu.cn/open-apis/im/v1/messages?receive_id_type=user_id";
private static final String AUTHORIZATION = "Bearer t-g1042h8JMSNCGICKKETF";
private static final String CONTENT_TYPE = "application/json";
public static void main(String[] args) {
try {
// Create and configure the connection
URL url = new URL(URL);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Authorization", AUTHORIZATION);
connection.setRequestProperty("Content-Type", CONTENT_TYPE);
connection.setDoOutput(true);
// Write the request body
String jsonBody = "{"
+ "\"content\": \"{\\\"text\\\":\\\"2-17\\\"}\","
+ "\"msg_type\": \"text\","
+ "\"receive_id\": \"abcd1234\"}";
try (OutputStream os = connection.getOutputStream()) {
byte[] input = jsonBody.getBytes("UTF-8");
os.write(input, 0, input.length);
}
// Read the response
try (BufferedReader br = new BufferedReader(new InputStreamReader(connection.getInputStream(), "UTF-8"))) {
StringBuilder response = new StringBuilder();
String responseLine;
while ((responseLine = br.readLine()) != null) {
response.append(responseLine.trim());
}
System.out.println("Response: " + response.toString());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}在Java11+中加入了新的特性,可以使用
HttpClient
进行访问,或者使用OkHttp
库。如果要用Springboot中调用相关方法,要注意是否要注册为Bean,具体可参考博客:。
二. SDK
也就是使用飞书官方实现封装号的包进行访问分别使用,Python、Java SDK来给出样例
现在以飞书机器人发送消息API的调用作为示例进行演示,注意此样例需要获取相应的app_id、app_secret和user_id,此样例中的两个参数是无法实际使用的。
下面是三种访问的代码:
Python SDK
需要先导入Python的包,包括
lark_oapi
和1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43import json
import lark_oapi as lark
from lark_oapi.api.im.v1 import *
# SDK 使用说明: https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/server-side-sdk/python--sdk/preparations-before-development
# 以下示例代码默认根据文档示例值填充,如果存在代码问题,请在 API 调试台填上相关必要参数后再复制代码使用
# 复制该 Demo 后, 需要将 "YOUR_APP_ID", "YOUR_APP_SECRET" 替换为自己应用的 APP_ID, APP_SECRET.
def main():
# 创建client
client = lark.Client.builder() \
.app_id("YOUR_APP_ID") \
.app_secret("YOUR_APP_SECRET") \
.log_level(lark.LogLevel.DEBUG) \
.build()
# 构造请求对象
request: CreateMessageRequest = CreateMessageRequest.builder() \
.receive_id_type("user_id") \
.request_body(CreateMessageRequestBody.builder()
.receive_id("789d7b34")
.msg_type("text")
.content("{\"text\":\"2-17\"}")
.build()) \
.build()
# 发起请求
response: CreateMessageResponse = client.im.v1.message.create(request)
# 处理失败返回
if not response.success():
lark.logger.error(
f"client.im.v1.message.create failed, code: {response.code}, msg: {response.msg}, log_id: {response.get_log_id()}, resp: \n{json.dumps(json.loads(response.raw.content), indent=4, ensure_ascii=False)}")
return
# 处理业务结果
lark.logger.info(lark.JSON.marshal(response.data, indent=4))
if __name__ == "__main__":
main()Java SDK
此方法事先要
oapi-sdk
依赖:1
2
3
4
5<dependency>
<groupId>com.larksuite.oapi</groupId>
<artifactId>oapi-sdk</artifactId>
<version>${feishu}</version>
</dependency>${feishu}
要导入你需要的版本号,具体可以去maven的网站看看。1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41import com.google.gson.JsonParser;
import com.lark.oapi.Client;
import com.lark.oapi.core.utils.Jsons;
import com.lark.oapi.service.im.v1.model.*;
import java.util.HashMap;
import com.lark.oapi.core.request.RequestOptions;
// SDK 使用文档:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/server-side-sdk/java-sdk-guide/preparations
// 复制该 Demo 后, 需要将 "YOUR_APP_ID", "YOUR_APP_SECRET" 替换为自己应用的 APP_ID, APP_SECRET.
// 以下示例代码默认根据文档示例值填充,如果存在代码问题,请在 API 调试台填上相关必要参数后再复制代码使用
public class CreateMessageSample {
public static void main(String arg[]) throws Exception {
// 构建client
Client client = Client.newBuilder("YOUR_APP_ID", "YOUR_APP_SECRET").build();
// 创建请求对象
CreateMessageReq req = CreateMessageReq.newBuilder()
.receiveIdType("user_id")
.createMessageReqBody(CreateMessageReqBody.newBuilder()
.receiveId("abcd1234")
.msgType("text")
.content("{\"text\":\"2-17\"}")
.build())
.build();
// 发起请求
CreateMessageResp resp = client.im().v1().message().create(req);
// 处理服务端错误
if(!resp.success()) {
System.out.println(String.format("code:%s,msg:%s,reqId:%s, resp:%s",
resp.getCode(), resp.getMsg(), resp.getRequestId(), Jsons.createGSON(true, false).toJson(JsonParser.parseString(new String(resp.getRawResponse().getBody(), StandardCharsets.UTF_8)))));
return;
}
// 业务数据处理
System.out.println(Jsons.DEFAULT.toJson(resp.getData()));
}
}
三. 其它
文档中还有很多接口的使用以及更多的案例,具体可以参考飞书开放平台开发文档。
- 标题: API调用(webhook/sdk)
- 作者: Sabthever
- 创建于 : 2025-02-17 14:57:10
- 更新于 : 2025-02-20 16:17:31
- 链接: https://sabthever.online/2025/02/17/technology/API调用/
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。